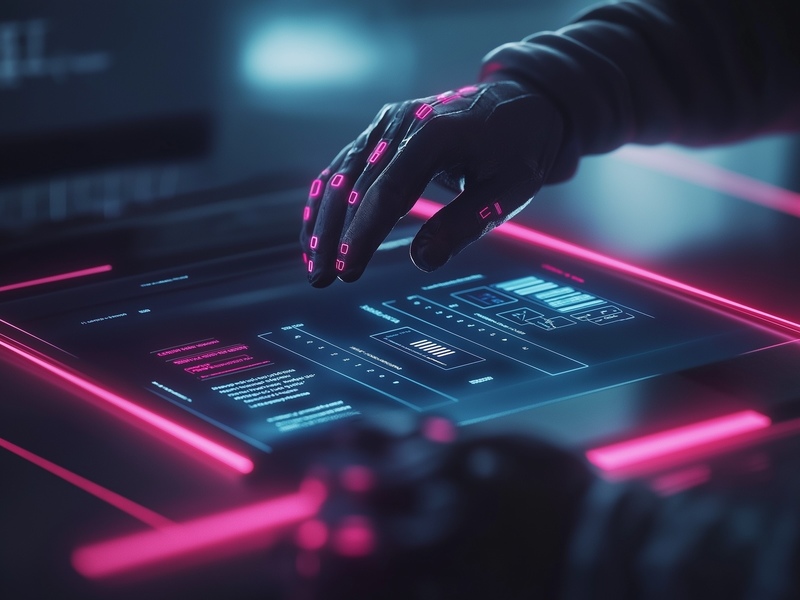
Available Platforms
Authentication made easy.
Sequence provides a wide variety of authentication options, from web2 to web3. All easily configurable and customizable for your brand.9 lines collapsed
10 <WalletConnectionDetail address={address} />
11 <button onClick={() => disconnect()}>Disconnect</button>
3 lines collapsed
15 <p>Not connected</p>
16 <button onClick={() => setOpenConnectModal(true)}>Connect</button>
3 lines collapsed
Not connected
Go ahead, try with your email or social to see the power of Sequence embedded wallets.
Sign a message
No popups, no modals. Seamless UX with a cross-platform, non-custodial wallet.1 import { useEffect } from "react";
2 import { useAccount, useSignMessage } from "wagmi";
3 import { WalletConnectionDetail } from "~/components/wallet-connection-detail/WalletConnectionDetail";
4 interface Props {
5 setData: (data: `0x${string}` | undefined) => void;
6 }
7 export const SignMessageWidget = (props: Props) => {
8 const { setData } = props;
9 const { address } = useAccount();
10 const { isPending, data, signMessage } = useSignMessage();
11 useEffect(() => setData(data));
12 return address ? (
13 <>
14 <WalletConnectionDetail address={address} />
15 {isPending ? (
16 <button className="ghost">Pending...</button>
17 ) : (
18 <button onClick={() => signMessage({ message: "hello" })}>
19 Sign "hello"
20 </button>
21 )}
22 {data ? (
23 <div className="flex flex-col gap-1 items-start px-12 py-1 mt-8">
24 <span className="text-14 opacity-75">Signature</span>
25 <p className="break-all font-mono text-12"> {data}</p>
26 </div>
27 ) : null}
28 </>
29 ) : (
30 <>
31 <p>Not connected</p>
32 </>
33 );
34 };
Connect a wallet test signing a message
Send a transaction
Built on top of wagmi for web, making integration and usage a breeze.1 import { useAccount, useSendTransaction } from "wagmi";
2 import { useEffect } from "react";
3 import { WalletConnectionDetail } from "~/components/wallet-connection-detail/WalletConnectionDetail";
4 interface Props {
5 setData: (data: `0x${string}` | undefined) => void;
6 }
7 export const SendTestTransactionWidget = (props: Props) => {
8 const { setData } = props;
9 const { address } = useAccount();
10 const { data, sendTransaction, isPending, error } = useSendTransaction();
11 useEffect(() => setData(data), [data, setData]);
12 return address ? (
13 <>
14 <WalletConnectionDetail address={address} />
15 {isPending ? (
16 <button className="ghost">Pending...</button>
17 ) : (
18 <button
19 onClick={() =>
20 sendTransaction({ to: address, value: BigInt(0), gas: null })
21 }
22 >
23 Send Transaction
24 </button>
25 )}
26 {data ? (
27 <div className="flex flex-col gap-1 items-start px-12 py-1 mt-8">
28 <span className="text-14 opacity-75">Transaction hash</span>
29 <p className="break-all font-mono text-12"> {data}</p>
30 </div>
31 ) : null}
32 {error && <p>{error?.message}</p>}
33 </>
34 ) : (
35 <>
36 <p>Not connected</p>
37 </>
38 );
39 };
Connect a wallet to send a transaction
Onboarding is only the beginning.
Check out the rest of the Sequence BluePrints to see how we enable everything from monetization to your backend.